Published on April 13, 2024 | Posted in Laravel
How to Update Input Values Dynamically based on another input with Laravel and Livewire
One of the things I love about modern development tools and frameworks is how they make it so easy to do simple features. Years ago, these sorts of things I’m about to show would be some jQuery gymnastic with a prayer that it works in IE. Updating another value based on input in Livewire is a simple process with modern frameworks like Livewire and Laravel.
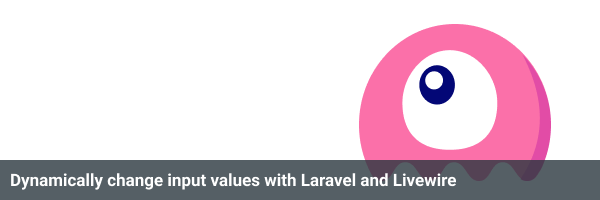
I will demonstrate how updating a specific field in Livewire dynamically changes the value of another field. Using a blog as an example, you automatically create the slug based on that title when you create a title.
I will assume that you have already created a component and added it to your view. In my case, I have a Laravel component called Post. In the Post template, we are going to add two Properties, one called title and the other called slug.
public $title;
public $slug;
Now go to the blade template with both the properties we created above. Are going to be added to the form with an input using wire:model attribute
<form class="space-y-5">
<div>
<label class="block mb-2" for="title">Title</label>
<input type="text" name="title" id="title" wire:model.live="title" />
</div>
<div>
<label class="block mb-2" for="slug">Slug</label>
<input type="text" name="slug" id="slug" wire:model="slug" />
</div>
</form>
With that setup, we will work on the reactive part of the form. Back to the Post.php template, we will use the updated method on the Lifecycle hook. So, the method runs every time our title property is updated.
What the code does below we look to see if the title property has been updated. If it has, we get the slug property using the Laravel slug helper and convert it into a URL.
public function updated($property)
{
if ($property == 'title') {
slug = Str::of($this->title)->slug;
}
}
Now, go back to the blade template, and we’re going to update the title tag so it changes live.
<input type="text" name="title" id="title" wire:model.live="title" />
Anytime you change the title input, the slug will get updated. It’s so impressive how Livewire makes this technique easy to do. However, making the change using live can result in many Network requests. My preferred method is Blur, which only runs when someone’s tabs are out of the field.
<input type="text" name="title" id="title" wire:model.blur="title" />
An alternative way would be to return to our Post.php file and change the updated function to updateTitle. Livewire is clever enough to know we are changing the title property, making the code much cleaner.
public function updatedTitle()
{
$this->slug = Str::of($this->title)->slug;
}
In summary, this is how you can reactively update another text input using the example of a blog title and post. I hope you found some use for this in your projects. Below is a video of the code above.